In our GameUiPlugin
we'll add a new scoreboard
system to handle updating the score display when the Game
score field is updated.
impl Plugin for GameUiPlugin {
fn build(&self, app: &mut App) {
app.add_startup_system(setup_ui)
.add_system(scoreboard);
}
}
The scoreboard system will read the Game
resource as well as query for the Text
component with a ScoreDisplay
component attached to it. This allows us to grab just the Text
we need to update.
Since there is only a single result in the query, we'll use a pattern we've seen before with the board query: .single()
. In this case we have a mutable reference to the Text
, so we need to use .single_mut()
instead.
Then it's a matter of setting the first section of the Text
to the game score. The game score isn't a string, so we convert it to a string first for display purposes.
fn scoreboard(
game: Res<Game>,
mut query_scores: Query<&mut Text, With<ScoreDisplay>>,
) {
let mut text = query_scores.single_mut();
text.sections[0].value = game.score.to_string();
}
We will also need to bring Game
into scope from the crate root.
use crate::{colors, FontSpec, Game};
That leaves us with an auto-updating score display.
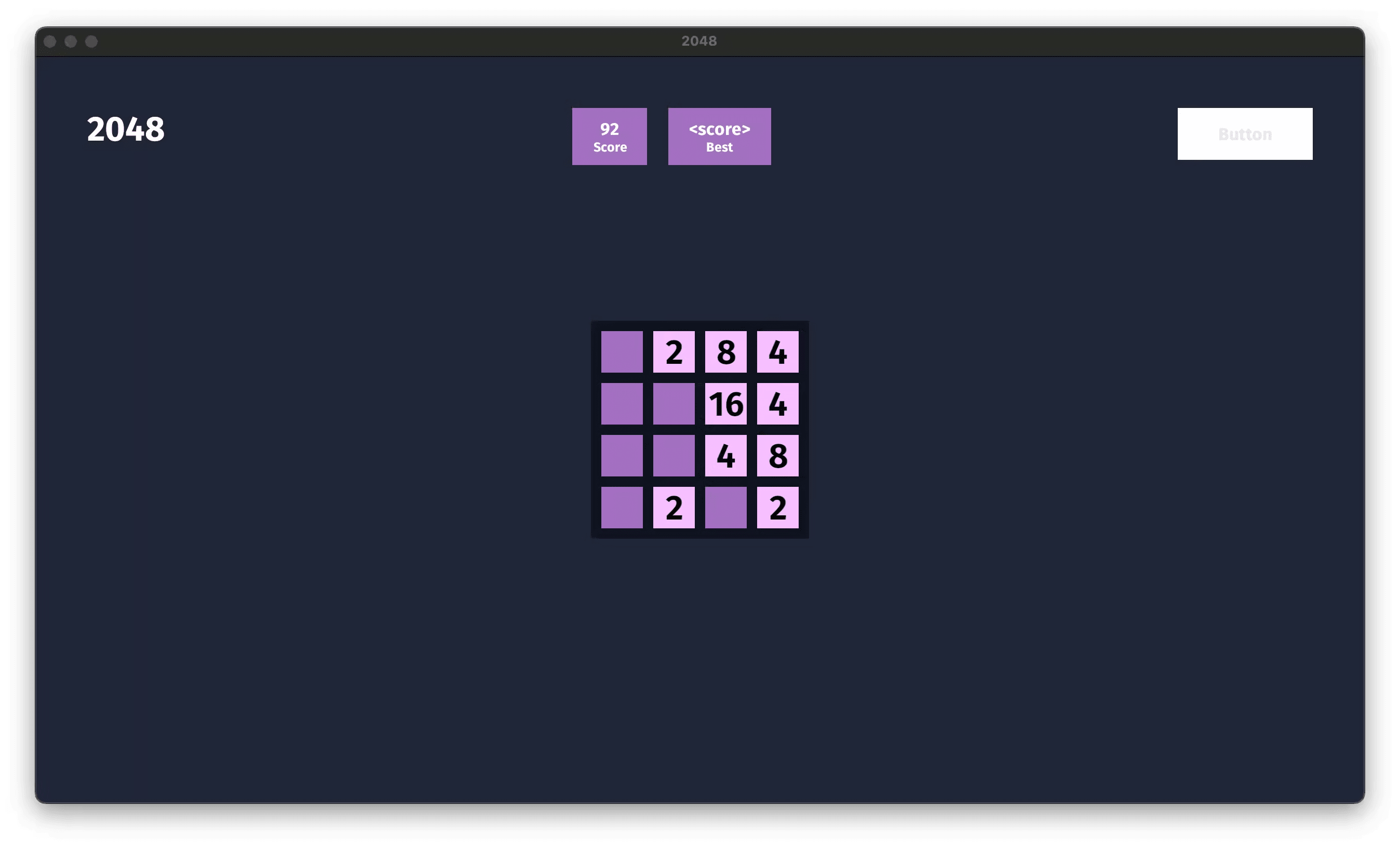