With a fresh new coat of paint for the board we probably want to change the background as well.
Our camera is responsible for painting all of the pixels we see. Any area of the screen that doesn’t have a sprite on it will be painted using a “clear color”.
We can configure what the ClearColor
is by using a Resource
which will get accessed and used by Bevy’s rendering system.
A Resource
is some data that isn’t associated with any entities or components.
fn main() {
App::new()
.insert_resource(ClearColor(
Color::hex("#1f2638").unwrap(),
))
.add_plugins(DefaultPlugins.set(WindowPlugin {
primary_window: Some(Window {
title: "2048".to_string(),
..default()
}),
..default()
}))
.add_startup_systems((setup, spawn_board))
.run()
}
This time we’ll use the hex
function on Color
to parse a hex code into a Color. Because this is parsing it could fail if we don’t give it a well formatted hex code, which is why the hex
function returns a Result<Color, HexColorError>
.
If we put in a hex code that doesn’t work we’d rather see our program crash in development, so we can .unwrap()
the Result here, which will return the parsed Color
for us.
We wrap the Color
in the ClearColor
struct so Bevy will find it when looking for the clear color, and insert it as a resource.
When we cargo run
now, we see the board in the middle of a blueish background.
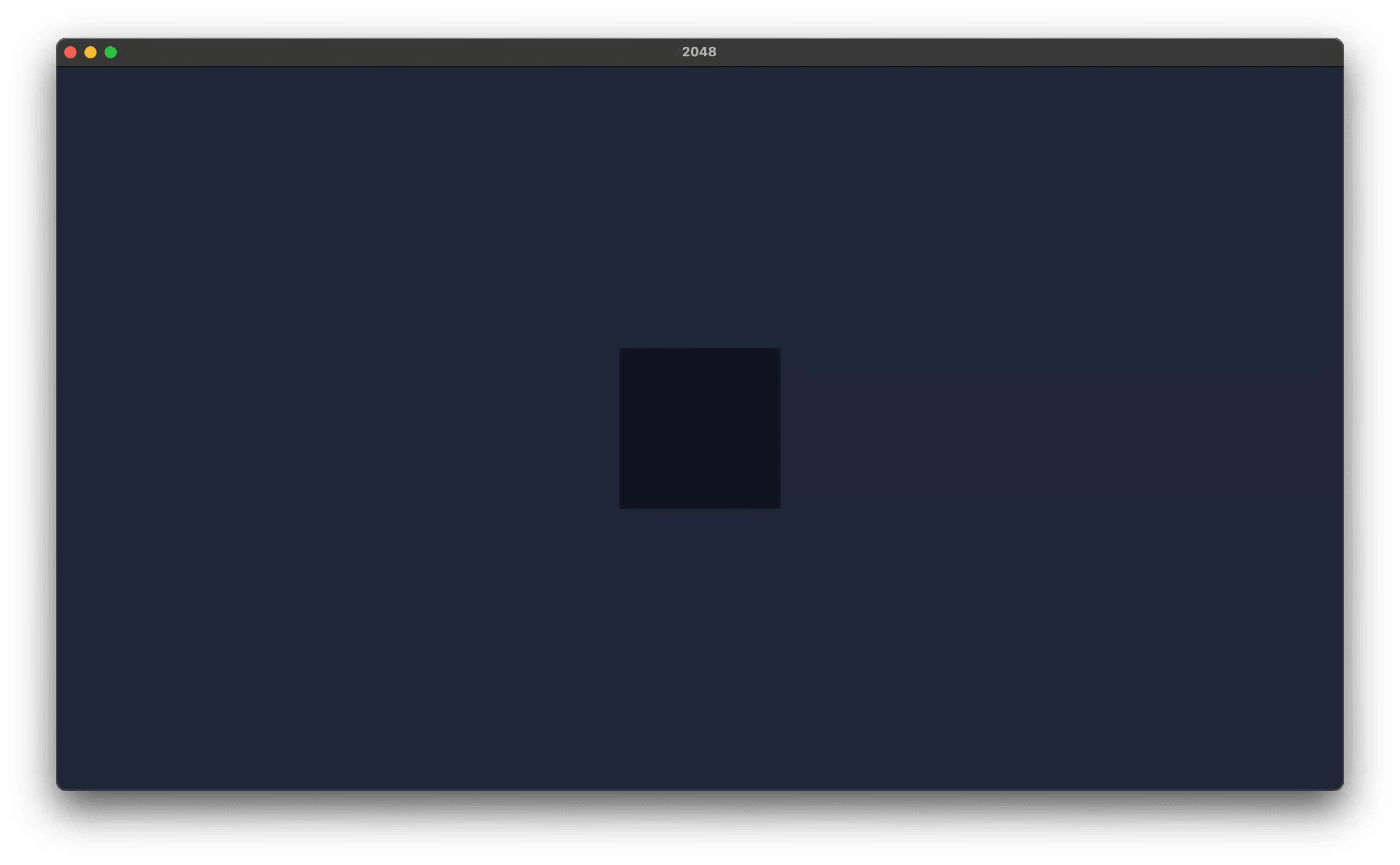