To start a new game after someone has already been playing we need to:
- Remove all current tiles on the board
- Place two new starter tiles on the board
- Start up all of the systems used to play the game
Removing all tiles on the board is something Bevy makes fairly direct. We can create a new game_reset
system that queries for all entities with a Position
component. If there are any, we need to despawn them, so we loop over the iterator to operate on each tile.
commands.entity
gives us an EntityCommands
builder that we can use to operate on the given entity. In this case Bevy has a despawn
and a despawn_recursive
method. despawn
will remove the current entity from the game, while despawn_recursive
will remove the current entity and all of it's children. In this case we want despawn_recursive
because we want to remove the Text
inside the tile as well.
fn game_reset(
mut commands: Commands,
tiles: Query<Entity, With<Position>>,
mut game: ResMut<Game>,
) {
for entity in tiles.iter() {
commands.entity(entity).despawn_recursive();
}
game.score = 0;
}
We'll additionally reset the Game
score to 0.
in src/main.rs
in the app builder we'll add this system as a new set tied to entering the RunState::Playing
state. This means that when we enter the Playing
state, the game_reset
system will run once, just like a startup system.
.add_system(
game_reset
.in_schedule(OnEnter(RunState::Playing)),
)
If you run the game now, you'll notice one big issue. The game_reset
system is running after the spawn_tiles
system, resulting in no tiles on the board when we start a new game!
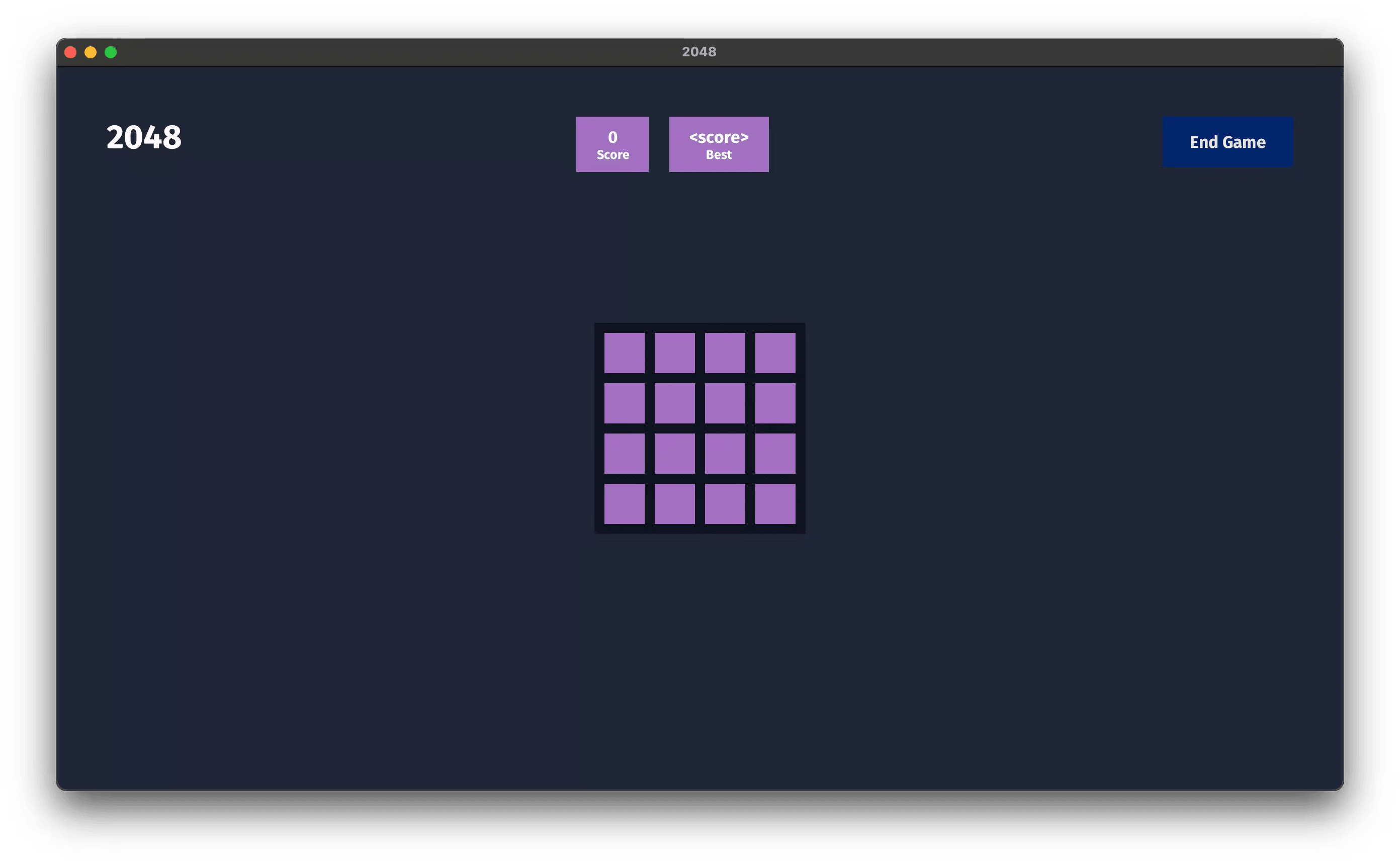
We can remove the spawn_tiles
system from the startup systems.
.add_startup_systems(
(setup, spawn_board, apply_system_buffers)
.chain(),
)
because we're going to add it after the game_reset
system now.
.add_systems(
(game_reset, spawn_tiles)
.in_schedule(OnEnter(RunState::Playing)),
)
This works!
Because of the way commands are applied (deferred and batched) and what we're changing in these systems, we can run both of these systems in parallel and the game_reset
system will only remove the pre-existing tiles while the spawn_tiles
system will only add new tiles to the board.
Running the game now will allow you to reset and restart the game.