While we can add a board and tiles to the screen, they won't be visible unless we set up a Camera first. To do that we'll bootstrap a new Camera2dBundle
using the defaults since 2048 is a 2d game.
fn setup(mut commands: Commands) {
commands.spawn(Camera2dBundle::default());
}
In addition to the concept of a Camera, there are three new ideas here: Bundles, Commands, and spawning.
Cameras
A Camera is an entity in Bevy with components attached to it. You can think of the Camera as almost exactly the same thing as a real DSLR digital camera, or the camera on your iPhone. A camera is responsible for determining quite a few aspects for how our app is rendered and for us all of the defaults work just fine.
Bundles
Bundles are ordered collections of components. They make it a bit easier to add lots of components at one time. For example, the Camera2dBundle
includes about 10 components we didn't have to set up or configure because we used the `default() method which gave us a Bundle of components that set up a 2d camera for us.
Commands and Spawning
In a Bevy game, basically everything is accessible through the World
struct, even though we mostly don’t see it. The World
struct stores and exposes operations on entities, components, and their associated metadata. Commands
are how we call those operations. In this example we use a command called spawn
to insert a new camera into our game.
Adding Systems
Now that we have a setup
function, we can use that as a system in our Bevy App. We only want the setup function to run once at startup because we only ever need one camera for our game, so we'll use .add_startup_system
on the App
.
use bevy::prelude::*;
fn main() {
App::new()
.add_plugins(DefaultPlugins.set(WindowPlugin {
primary_window: Some(Window {
title: "2048".to_string(),
..default()
}),
..default()
}))
.add_startup_system(setup)
.run()
}
fn setup(mut commands: Commands) {
commands.spawn(Camera2dBundle::default());
}
We can pass a function directly into add_startup_system
and Bevy will turn our function into a system for us.
Bevy turning out function into a system is how our function gets it's Commands
argument. Bevy understands from the type signature what our system needs, and makes sure that is available when we want to run it. We will use this functionality later to do more interesting queries for our systems.
If we cargo run
now, we’ll see that the background color of our app changed to a gray color. This is how we know the camera is working.
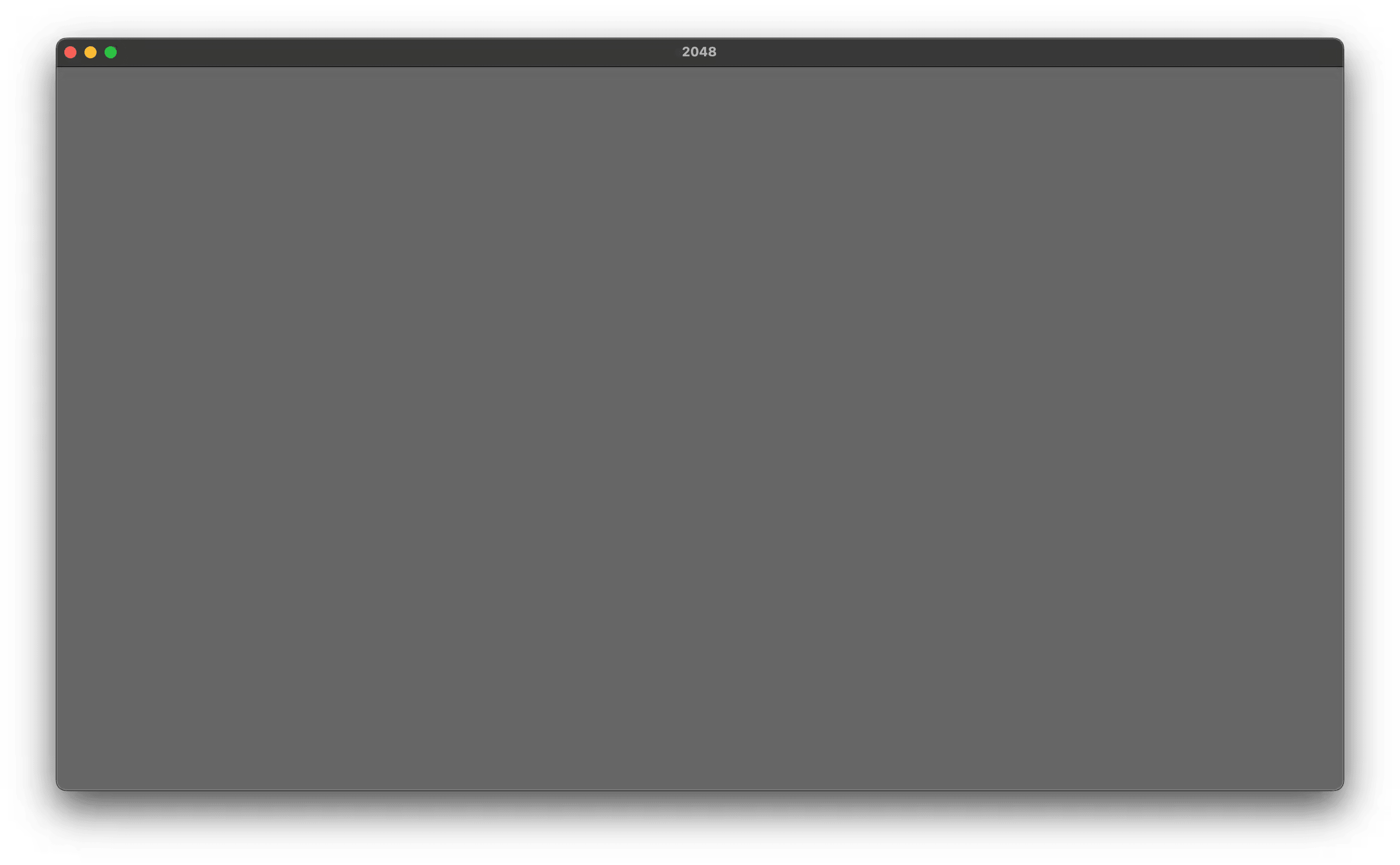